Hi there,
I have an combo box (Not the datagridviewcombobox) which has all the xml.file data label like this: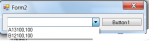
And this is my xml.file:
Then I also do have a datagridview which is empty. But as you can see the
The button 1 it contain of:
So my question now is that, how to I actually add the correct selected item from combobox into the datagridview which will then put 'A' into the fontstyle column, '12' into fontsize column and '100,100' into "location"?
THANK YOU
I have an combo box (Not the datagridviewcombobox) which has all the xml.file data label like this:
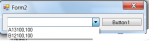
And this is my xml.file:
VB.NET:
<?xml version="1.0" standalone="yes"?>
<programsetting>
<panel>
<fontstyle>A</fontstyle>
<fontsize>13</fontsize>
<location>100,100</location>
</panel>
<panel>
<fontstyle>B</fontstyle>
<fontsize>12</fontsize>
<location>100,100</location>
</panel>
<test>
</programsetting>
Then I also do have a datagridview which is empty. But as you can see the
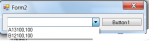
The button 1 it contain of:
VB.NET:
Dim col As New DataGridViewTextBoxColumn() Dim colsize As New DataGridViewTextBoxColumn()
Dim colloc As New DataGridViewTextBoxColumn()
Dim dgvRow As New DataGridViewRow
MainForm.dgv.Rows.Clear()
MainForm.dgv.Columns.Clear()
col.Name = ("fontstyle")
MainForm.dgv.Columns.Add(col)
colsize.Name = ("fontsize")
MainForm.dgv.Columns.Add(colsize)
colloc.Name = ("location")
MainForm.dgv.Columns.Add(colloc)
MainForm.dgv.Rows.Add(Me.ComboBox1.SelectedItem())
So my question now is that, how to I actually add the correct selected item from combobox into the datagridview which will then put 'A' into the fontstyle column, '12' into fontsize column and '100,100' into "location"?
THANK YOU
Last edited by a moderator: