BleepyEvans
Active member
- Joined
- May 2, 2011
- Messages
- 41
- Programming Experience
- 1-3
Hi guys, ive been working on a stopwatch project as some of you know.
Ive created the stopwatch, and thanks to some of your members, I finally got the stopwatch to add the timings into a datagridview every time the lap button is pressed.
Although because im converting the times to strings im having trouble trying to get the smallest value for the fastest lap.
Here what some of my form looks like:
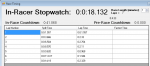
And some of my code:
Any ideas guys?
Ive created the stopwatch, and thanks to some of your members, I finally got the stopwatch to add the timings into a datagridview every time the lap button is pressed.
Although because im converting the times to strings im having trouble trying to get the smallest value for the fastest lap.
Here what some of my form looks like:
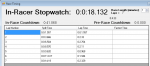
And some of my code:
Private Sub LapButton_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles LapButton.Click i += 1 If DataGridView1.Rows.Count > 0 Then DataGridView1.Rows.Add(i, StopwatchLabel.Text, laptimerer.Text) Else DataGridView1.Rows.Add(i, StopwatchLabel.Text, StopwatchLabel.Text) End If Label3.Text = Label3.Text + Val(1) lapTime = DateTime.Now End Sub
Private Sub StartButton_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles StartButton.Click startTime = DateTime.Now StartStopWatch() End Sub
Public Sub StartStopWatch() startTime = DateTime.Now StopWatch.Start() InRaceCountDownTime = DateTime.Now.AddMinutes(racelength.Text) InRaceCountDownTimer.Start() ProgressBar1.Value = 0 lapTime = DateTime.Now Lap.Start() End Sub
Private Sub Lap_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Lap.Tick Dim span As TimeSpan = DateTime.Now.Subtract(lapTime) laptimerer.Text = span.Minutes.ToString & ":" & span.Seconds.ToString & "." & span.Milliseconds End Sub
Any ideas guys?